Swift Enum in Objective-C is a crucial topic for developers working across both Swift and Objective-C ecosystems. Whether you're transitioning from Objective-C to Swift or integrating Swift components into an existing Objective-C project, understanding how Swift enums interact with Objective-C is essential. This article dives deep into the intricacies of Swift enums, their compatibility with Objective-C, and best practices for seamless integration. By the end of this guide, you'll have a clear understanding of how to leverage Swift enums in Objective-C projects effectively.
Swift enums are a powerful feature of the Swift programming language, offering a type-safe way to define a group of related values. However, Objective-C, being a more dynamic language, does not natively support Swift enums in the same way. This creates challenges when developers need to bridge the two languages. Fortunately, Swift provides mechanisms to make enums accessible in Objective-C, ensuring interoperability between the two languages. In this article, we'll explore these mechanisms and provide practical examples to help you master this topic.
The demand for interoperability between Swift and Objective-C has grown as more developers adopt Swift for new projects while maintaining legacy Objective-C codebases. This guide is designed to help you navigate the complexities of Swift enums in Objective-C, ensuring that your code remains clean, efficient, and maintainable. Whether you're a seasoned developer or just starting, this article will equip you with the knowledge and tools to handle Swift enums in Objective-C confidently.
Read also:Amiyah Scott The Inspiring Journey Of A Transgender Actress And Advocate
Table of Contents
- Introduction to Swift Enums
- Swift Enum Compatibility with Objective-C
- Bridging Swift Enums to Objective-C
- Using NS_ENUM and NS_OPTIONS
- Practical Examples of Swift Enums in Objective-C
- Limitations and Workarounds
- Best Practices for Using Swift Enums
- Common Mistakes to Avoid
- Real-World Use Cases
- Conclusion
Introduction to Swift Enums
Swift enums, short for enumerations, are a first-class type in Swift that allow you to define a common type for a group of related values. Enums make your code more readable, maintainable, and less error-prone by providing a clear and concise way to represent a finite set of options. For example, you might use an enum to represent the days of the week, states of a network request, or different types of errors.
Swift enums come with several powerful features that set them apart from traditional enums in other languages. These include:
- Associated Values: Enums can store additional information alongside each case.
- Raw Values: Enums can be backed by a raw value, such as an integer or string.
- Methods and Properties: Enums can have computed properties and methods, making them highly versatile.
Here's a simple example of a Swift enum:
enum NetworkResponse { case success(data: Data) case failure(error: Error) }
Associated Values in Swift Enums
Associated values allow Swift enums to carry additional data with each case. This feature is particularly useful when you need to represent more complex scenarios. For instance, in the NetworkResponse
enum above, the success
case carries the data received from the network, while the failure
case carries the error encountered.
Swift Enum Compatibility with Objective-C
One of the challenges developers face when working with Swift enums in Objective-C is that Objective-C does not natively support Swift enums. This limitation arises because Objective-C lacks the type safety and advanced features of Swift enums. However, Swift provides mechanisms to bridge enums to Objective-C, ensuring compatibility between the two languages.
To make a Swift enum accessible in Objective-C, you need to use the @objc
attribute. This attribute tells the Swift compiler to generate Objective-C-compatible code for the enum. However, not all Swift enums can be bridged to Objective-C. Only enums with raw values (such as integers or strings) and those marked with @objc
are compatible.
Read also:Rob Kardashian A Comprehensive Look Into His Life Career And Influence
Here's an example of a Swift enum marked with @objc
:
@objc enum Day: Int { case monday = 1 case tuesday = 2 case wednesday = 3 }
Limitations of @objc Enums
While the @objc
attribute enables interoperability, it comes with limitations:
- Enums with associated values cannot be marked with
@objc
. - Only enums with raw values of type
Int
orString
are supported. - Objective-C enums lack the advanced features of Swift enums, such as computed properties and methods.
Bridging Swift Enums to Objective-C
Bridging Swift enums to Objective-C involves using the @objc
attribute and ensuring the enum adheres to Objective-C's constraints. When you mark an enum with @objc
, the Swift compiler generates an Objective-C-compatible representation of the enum. This allows you to use the enum in Objective-C code seamlessly.
Here's how you can bridge a Swift enum to Objective-C:
@objc enum StatusCode: Int { case success = 200 case notFound = 404 case serverError = 500 }
In Objective-C, you can access the enum as follows:
StatusCode statusCode = StatusCodeSuccess; NSLog(@"Status Code: %ld", (long)statusCode);
Using Raw Values for Bridging
Raw values are essential for bridging Swift enums to Objective-C. By assigning raw values to each case, you ensure that the enum can be represented in Objective-C. The raw values can be of type Int
or String
, depending on your requirements.
Using NS_ENUM and NS_OPTIONS
When working with Objective-C, you may encounter NS_ENUM
and NS_OPTIONS
. These macros are used to define enums in Objective-C and are compatible with Swift. NS_ENUM
is used for standard enums, while NS_OPTIONS
is used for bit masks.
Here's an example of an NS_ENUM
in Objective-C:
typedef NS_ENUM(NSInteger, Color) { ColorRed, ColorGreen, ColorBlue };
In Swift, you can access this enum as follows:
let color: Color = .red
NS_OPTIONS for Bit Masks
NS_OPTIONS
is used when you need to represent multiple options using bitwise operations. This is common in scenarios like setting flags or options for a method.
Practical Examples of Swift Enums in Objective-C
Let's explore some practical examples of using Swift enums in Objective-C. These examples will demonstrate how to bridge enums, handle raw values, and work with Objective-C-compatible enums.
Example 1: Bridging a Simple Enum
Consider a Swift enum representing HTTP methods:
@objc enum HTTPMethod: Int { case get = 1 case post = 2 case put = 3 case delete = 4 }
In Objective-C, you can use this enum as follows:
HTTPMethod method = HTTPMethodGET; NSLog(@"HTTP Method: %ld", (long)method);
Limitations and Workarounds
While Swift enums are powerful, their use in Objective-C comes with limitations. For example, enums with associated values cannot be bridged to Objective-C. To work around this, you can use structs or classes to represent the associated data.
Workaround for Associated Values
Instead of using an enum with associated values, you can define a struct to encapsulate the data:
struct NetworkResponse { let success: Bool let data: Data? let error: Error? }
Best Practices for Using Swift Enums
To ensure your Swift enums are effective and compatible with Objective-C, follow these best practices:
- Use raw values for enums that need to be bridged to Objective-C.
- Mark enums with
@objc
only when necessary. - Avoid using associated values in enums that need Objective-C compatibility.
Common Mistakes to Avoid
Here are some common mistakes developers make when working with Swift enums in Objective-C:
- Using associated values in enums marked with
@objc
. - Forgetting to assign raw values to enum cases.
- Overusing
@objc
for enums that don't need Objective-C compatibility.
Real-World Use Cases
Swift enums in Objective-C are commonly used in scenarios like:
- Defining error codes for network requests.
- Representing states in a state machine.
- Handling user actions in a UI framework.
Conclusion
In this article, we've explored the intricacies of Swift enums in Objective-C, including their compatibility, bridging mechanisms, and best practices. By understanding how to bridge Swift enums to Objective-C and leveraging tools like NS_ENUM
and NS_OPTIONS
, you can ensure seamless interoperability between the two languages.
We encourage you to experiment with the examples provided and apply these concepts to your projects. If you have any questions or insights, feel free to leave a comment below. Don't forget to share this article with fellow developers and explore other resources on our site for more in-depth guides. Happy coding!
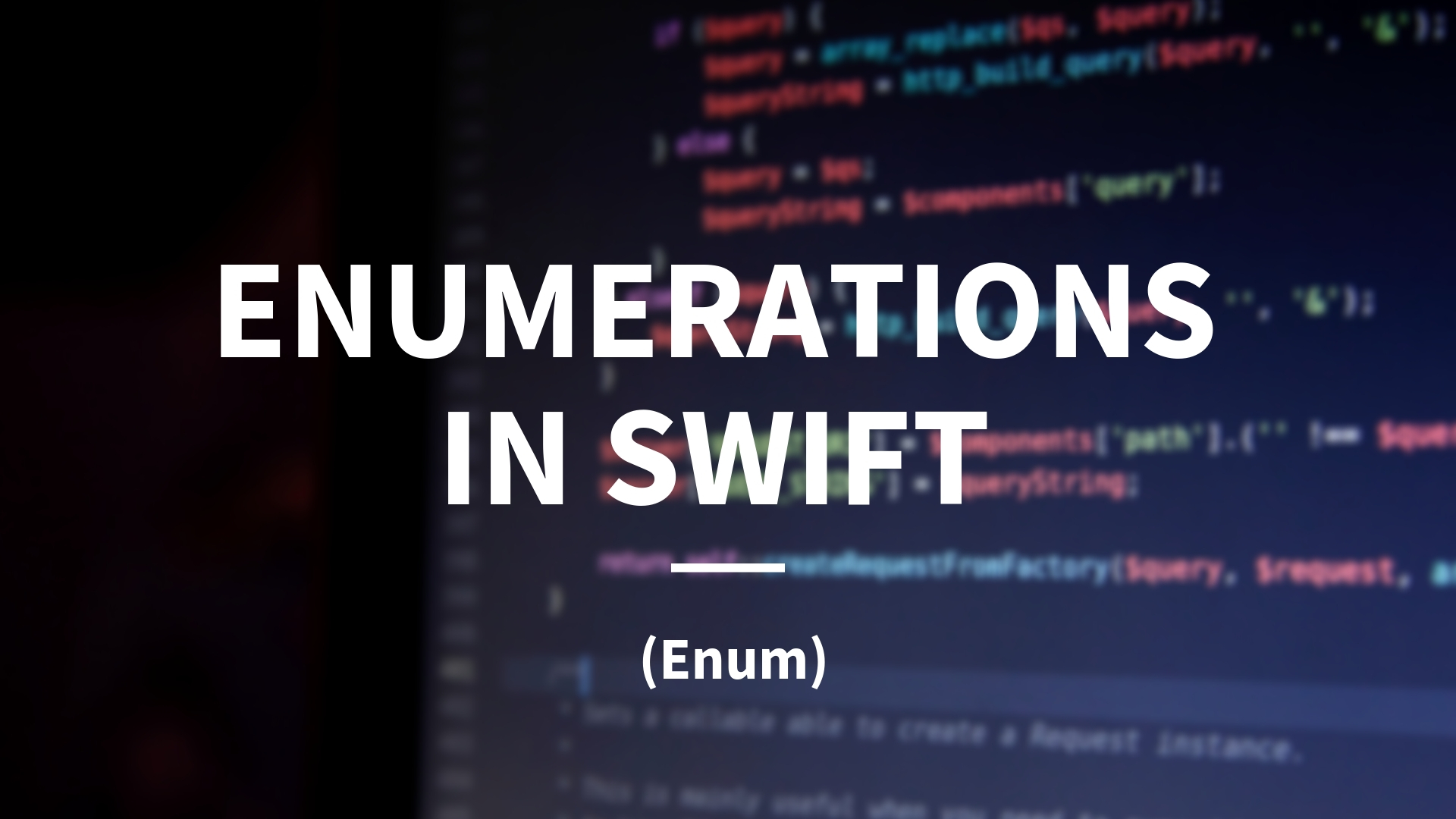
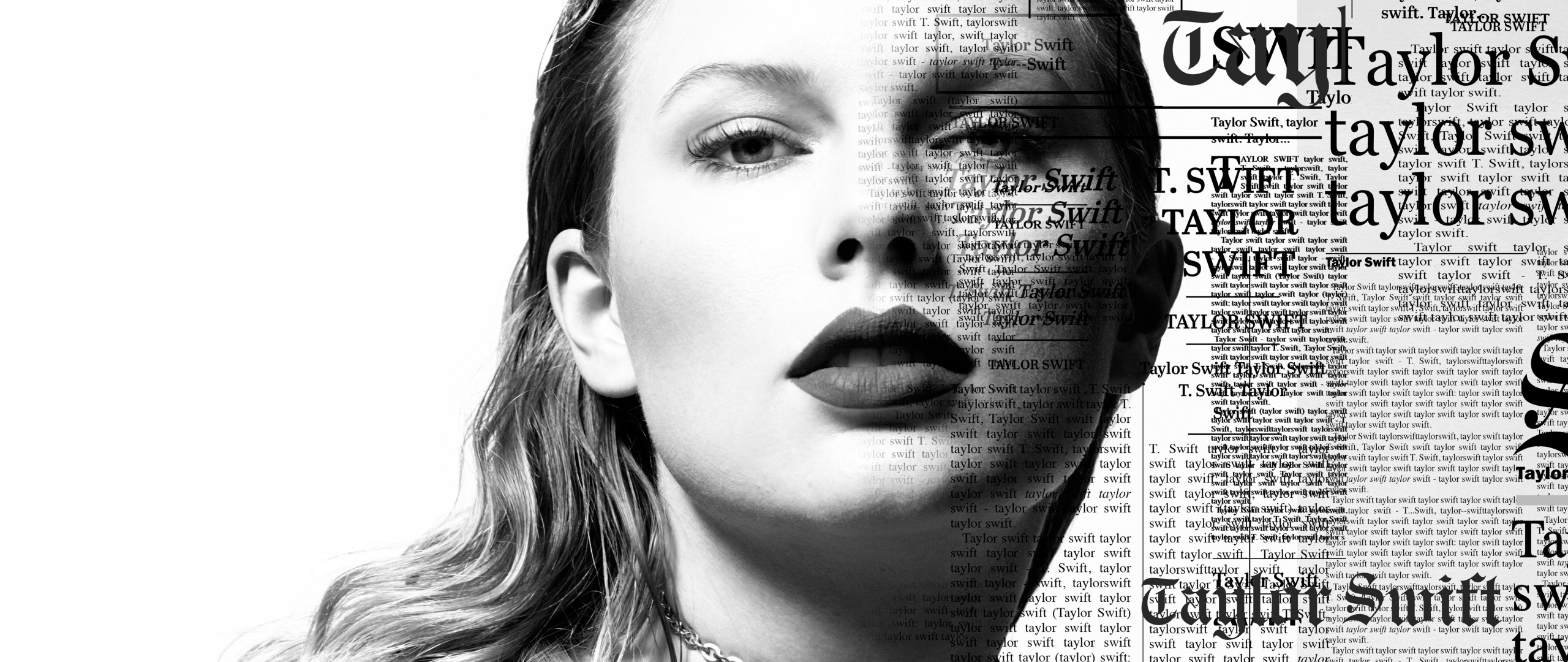